Author: Manuel Lemos
Viewers: 889
Last month viewers: 4
Categories: PHP Tutorials, Sponsored
Wix is a platform for publishing Web sites very quickly. Now Web site owners can develop Wix Web site features faster using Wix code.
Read this tutorial to learr more about Wix Code and how you can your own create Web sites faster using JavaScript.

Why Wix Code Uses JavaScript
Wix Code gives you the ability to add your own code to a Wix site. We chose JavaScript as the language we support both in both the front-end and the backend, and as the language of our APIs.
JavaScript in the Front-end
"Any application that can be written in JavaScript, will eventually be written in JavaScript."
Atwood's Law, Jeff Atwood
Wix Code lets you add your own custom JavaScript to control how the front-end of your site behaves. We chose JavaScript because it is arguably the standard language for front-end web development.
JavaScript lets you add behaviors to your site that respond to user actions, without calling the server or loading a new page. This lets you implement element animations that give your site a more engaging UX.
You can also add logic to your site like client-side validation, which gives your users a more immediate response to their input.
JavaScript in the Backend with Node.js
"Node.js enables JavaScript to be used for server-side scripting" Consequently, Node.js has become one of the foundational elements of the 'JavaScript everywhere' paradigm, allowing web application development to unify around a single programming language, rather than rely on a different language for writing server-side scripts."
Node.js is a strong contender as the standard for using JavaScript in the backend. We decided to use Node.js in our backend. This means that you only have one language to learn to completely customize your site’s functionality both front-end and backend.
Earlier we mentioned using JavaScript to create client-side validation in your site. While you might add client-side validation to make your site respond more quickly to user actions, you may still want to run a validation check in the backend before actually submitting data to your database. Since you use JavaScript in your backend, you can now reuse your front-end code in the backend wherever you need to.
Working with JavaScript in Wix Code
Wix Code gives you everything you need to get up and coding in your site, including both a built-in IDE and hassle-free backend, and an easy way to let your front-end and backend communicate.
Built-in IDE
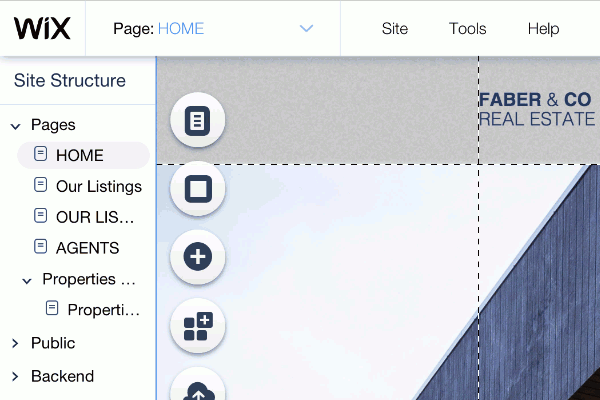
Wix Code includes a built-in online IDE that makes it easy to add code to your site with zero setup. This IDE works for your front-end files and lets you add page-specific code or code that you want to run on your entire site. You can also use the IDE to add code to backend files like data hooks, custom routers, web modules, and HTTP functions, or any other files you need.
The IDE makes coding easy because all your code is automatically integrated with your site. The IDE also includes professional tools to make coding easier, like code completion for elements and their properties or methods (type Ctrl + space after our $ selector or after a selected element). Along the way, the IDE also provides JSLint feedback to help you code using best practices.
To make debugging easier, any messages you log to your console in the front-end are displayed when you preview your site in the Developer Console in Wix’s Preview mode. Logs in backend code can be seen in your browser’s developer tools console as well.
Built-in Backend
Wix Code gives you a built-in backend. That means that you don't have to worry about creating, managing, and monitoring a backend infrastructure. We take care of setting up and provisioning the servers and monitoring their performance. We also give you built-in database functionality that you can use with or without our wix-data API.
Most importantly, we give you web modules: an easy way to let your front-end code call your backend code.
Web Modules
Web modules enable you to write functions that run server-side in the backend, which you can then easily call in your front-end code. Wix Code handles all the client-server communication required to enable this access.
To help with debugging, you can log messages to the console in web module code. These logs are displayed in the browser’s console.
Web modules also have permissions settings, so you can be sure that no one can access or use your backend code in ways that you didn't intend, either through your site's functionality or using a browser's developer tools.
In case you're curious, here's what's going on behind the scenes. When you import a web module on the client-side, you get a proxy function to the web module function. This proxy function uses an XMLHttpRequest to invoke the function in the backend. The runtime listens to those invocations and calls the appropriate function. The arguments and return value are serialized using JSON.
Unlike regular modules that allow you to export functions, objects, and other items, you can only export functions from web modules. Web modules also always return a promise. This is true even if, in the implementation of the function, it returns a value. For example, if your web module function returns a value, like this:
// Filename: aModule.jsw (web modules need to have a *.jsw* extension)
export function multiply(factor1, factor2) {
return factor1 * factor2;
}
When you call the function, it still returns a promise that resolves to the value. So you need to use it like this:
import {multiply} from 'backend/aModule';
multiply(4,5).then(function(product) {
console.log(product);

});
// Logs: 20
JavaScript Standards Support
Wix Code supports writing code using the latest JavaScript standards. You can write code using ES2015 syntax both in the backend and the front-end, including:
Promises
async/await for working with Promises
Support for the new modules
Arrow functions
Destructuring assignments
let and const declarations
Browsers are gradually adopting the new JavaScript standards. But you don’t have to worry about which browsers will understand you code. Until the new JavaScript standards are fully implemented, Wix Code transpiles your code into ES5, so it can run in current browsers.
Wix Code also supports source maps, so even though the browser runs transpiled ES5 code, you can debug your ES2015 source code in your browser's developer tools.
Optimization
Wix Code also takes care of efficiently delivering your code to the browser. Your code is minified and source files are combined (bundled) without you having to configure anything.
Code Examples
Wix Code offers a number of APIs that let you control your site’s functionality, including APIs for our database and custom routers, and for exposing your site’s functionality as a service, among others.
The $w API is used to work with the elements on your site’s pages. For example, here we create an onClick event handler for a button. The handler shows or hides an image based on the image's current state. It also changes the label of the button accordingly.
$w.onReady( () => {
$w("#showHideButton").onClick( (event, $w) => {
if( $w("#myImage").hidden ) {
$w("#myImage").show();
event.target.label = "Hide";
}
else {
$w("#myImage").hide();
event.target.label = "Show";
}
} );
} );
The wix-fetch API is used for making HTTP requests to 3rd party services. For example, here we have a backend function that receives the name of a city. It sends a request to a weather API to get the weather in that city. When a response is received, the function returns the current temperature to the calling function.
import {fetch} from 'wix-fetch';
export function getCurrentTemp(city) {
const url = 'https://api.openweathermap.org/data/2.5/weather?q=';
const key = '<api key placeholder>';
let fullUrl = url + city + '&appid=' + key + '&units=imperial';
return fetch(fullUrl, {method: 'get'})
.then(response => response.json())
.then(json => json.main.temp);
}
Learn More
The ability to add JavaScript to either your front-end or backend means that you can build a Wix site in entirely new ways.
For more information about the advanced features Wix Code offers, see our Resource Center.
You need to be a registered user or login to post a comment
1,616,870 PHP developers registered to the PHP Classes site.
Be One of Us!
Login Immediately with your account on:
Comments:
No comments were submitted yet.